Angular JS: Sharing data between child and parent components
Introduction
Angular is one of the widely used JavaScript frameworks, written in typescript. It is a component-based framework which is used for developing SPAs and large enterprise applications. It was developed by Google in 2009, and as a result, there have been many iterations. In general, frameworks increase performance and web development efficiency by providing a consistent structure for developers. It also offers developers a host of additional features which can be added to the software/application without any additional effort, hence saving a lot of development time.
Benefits
- Faster Development Process: It allows the developer to build a highly efficient application faster with the help of technical advantages that the angular framework provides like – Angular CLI, Two-Way data binding, built-in routing, templating and a large developer community which makes angularJS web development easier.
- Lightweight web Apps: Earlier performance of large-scale applications was very poor. Thanks to angular for the Lazy-Load feature. Which significantly improves the performance of such large-scale apps by reducing the size of the initially-loaded app.
- Efficient Problem-solving patterns: It offers an efficient design pattern, dependency injection and service which helps in solving productivity issues and speeding up the development process.
- Cross-Platform Development: It also supports cross-platform development. It allows the developers to write code that can run on multiple platforms like mobile and web applications.
Sharing data between Parent & Child components
Data sharing between components in angular is an important thing in a component-based framework. Small components are good to manage in angular. When we start breaking down the complex requirements into smaller ones (I.e., smaller components) then it's very important to have a proper data-sharing mechanism. There are multiple ways in which data is shared between the components. Few of them are
- Parent-to-child component
- Child to a parent component
- Sharing data between sibling components
Let us see how this can be achieved
Let us consider the below hierarchy for understanding the purpose
<parent-component>
<child-component></child-component>
</parent-component>
Here <parent-component> serves as a context for the <child-component>
@Input() and @Output() decorators give the child component a way to communicate with the parent component
@Input(): Lets the parent component pass the data to the child component
@Output(): Using this decorator child component can pass data to the parent component
Sending data from Parent to Child Component
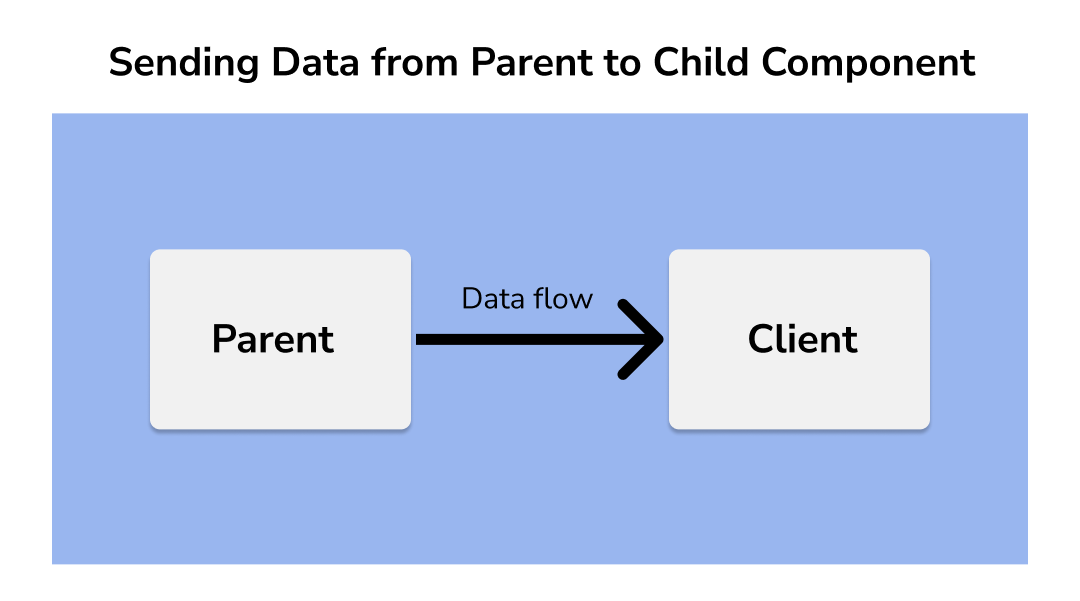
- Child Component
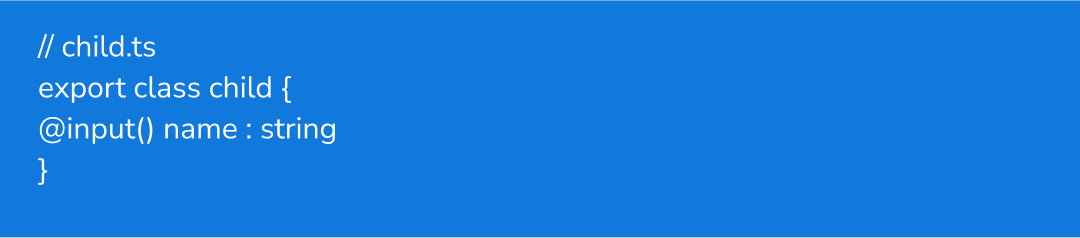
Create a child component to receive the input from the parent component
@Input() decorator in the child component signifies that it can receive data from the parent component. @Input() properties can have any type, such as string, number, object etc
- Binding the Property in the Parent
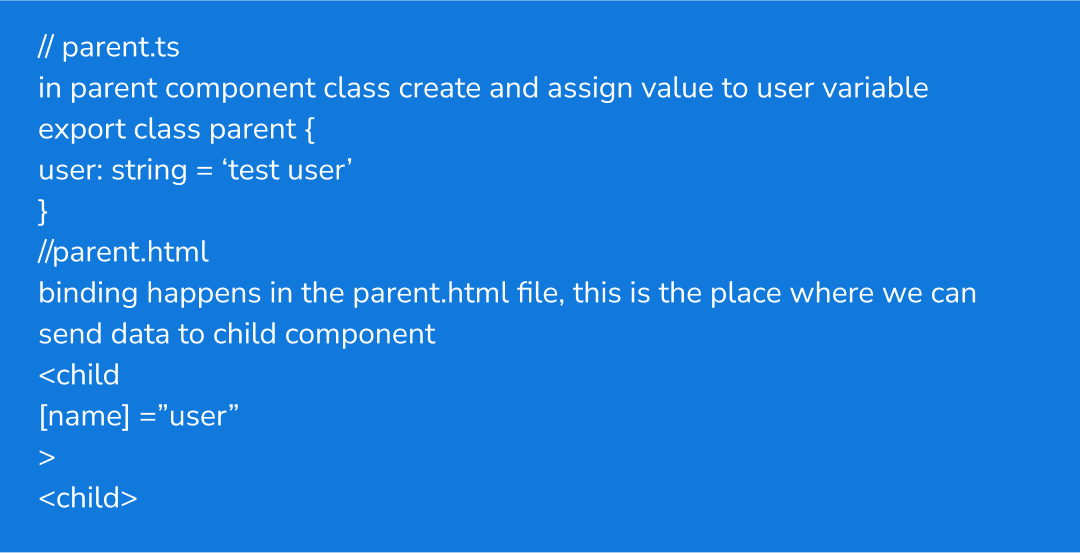
- Use the property in the child component
// child.html
Now the data will be available in the child component. By using interpolation binding syntax we can access the value in the template file.
<p> User: {{name}} </p>
Sending data from child to parent
For sharing data from child to parent we need an output decorator.
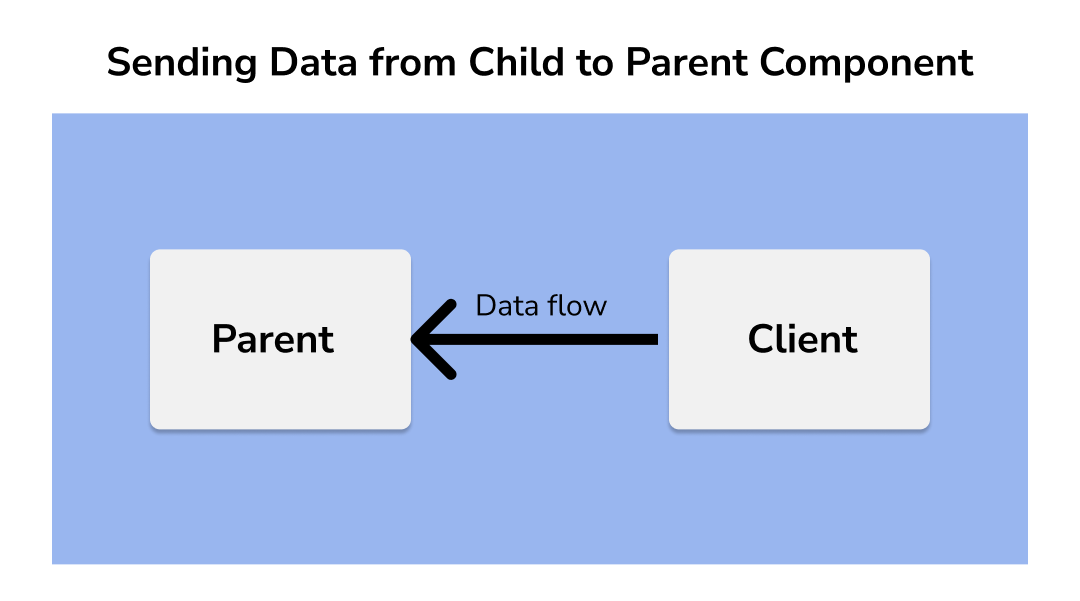
@Output() decorator allows data to be sent from the child component to the parent component. This decorator acts as a doorway for the transfer of data. The child component needs to notify the parent component about the change/update in data. This can be done by using event emitters.
- Preparing the child component for emitting data
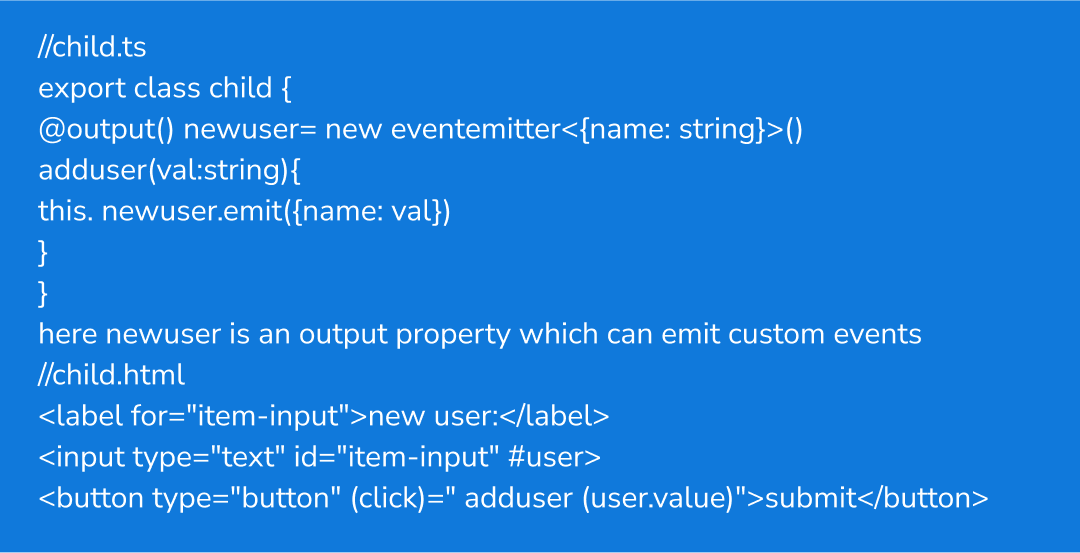
Whenever the user clicks Submit button, an event is emitted. Which contains the new user’s name.
The next step is to instruct the child selector in the parent component to listen to this event. This can be done by event binding.
- Configuring the parent component to access the data from the child

Now the parent component is aware of the event but we have to create a method to access the data that the child component is emitting.
//parent.ts
In the parent component, let us create a class with a variable to store the data and a method that will be invoked whenever the child component emits an event.
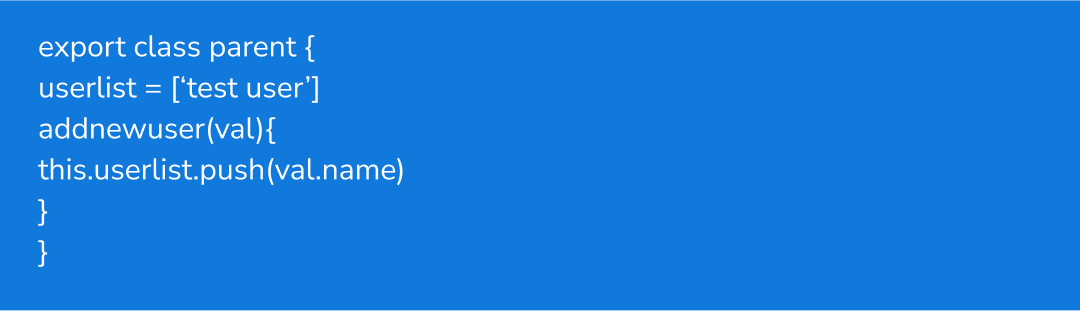
Sharing data between sibling components
This can be achieved by sharing the data from one child to parent by using an output decorator and event emitter. Once the data is received in the parent component, we can share it with other child components using the input decorator. To achieve this we have to follow the first two steps.