How to add Authentication and route guard to Vue App Using Firebase?
What is Firebase?
Firebase is a platform developed by Google used to create mobile and web applications. It was originally an independent company founded in 2011.
Firebase provides a very simple and quick way to add authentication to your Vue.js application. In this article, I will show you how easy it is to allow users to register with your application using their email and password.
In 2014, Google acquired the platform and it is now their flagship offering for app development. Cloud Storage for Firebase is a powerful and cost-effective storage service. The Cloud Storage SDK supports file uploads and downloads for your apps. You can use the cloud storage SDK to store images, audio, video, or other user-generated content.
Prerequisites
When building any type of application, privacy and security are two important factors to keep in mind. With cyber criminals becoming more advanced, authentication is an important step to deter hacking and protect your users’ most valuable information.
Firebase Authentication provides backend services to help authenticate users in your application, supporting different authentication methods, like passwords and phone numbers, and supporting identity providers including Google, Twitter, and Facebook.
To integrate Firebase Authentication into your application, you can use either the Firebase UI, which handles the UI flows for signing users in with different providers, or you can manually set up the Firebase SDK in your project and configure support for whatever provider you would like to use.
Overview
- How to Create our Project
- How to Add New Components
- Create Login and Sign-up Form
- Update the Routes
- Register New User
- Login Users
- Create a Route Guard
- Logout Users
- Conclusion
How to Create a Project?
To get started, we’ll use the Vue CLI v3.0 to quickly scaffold a new Vue project. Then, install the new CLI globally by running the following command:
npm install -g @vue/cli
Next, create a new Vue project by running the command below:
vue create firebase-auth
Next, create a project in firebase, and enable the authentication service. To install a firebase into your Vue project, please run the command below,
npm install firebase –save
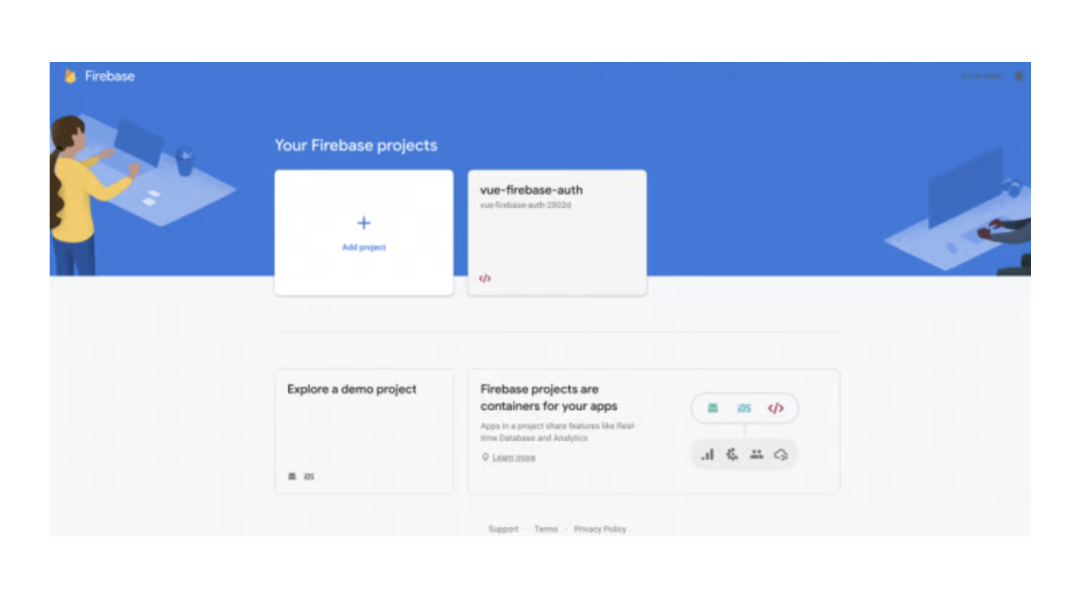
How to Add New Components?
When we created our application with Vue Router, it automatically created two routes for our application — Home and About. We will use Home as the login for our application. We will use the About page to register as a new user for our application.
When a registered user logs in to our application, we want to show them the dashboard page. We also want to provide a way for a user to log out of our application. Currently, neither of these options is available in our application so let’s add them now.
Open up the App.vue file. Currently, the nav has two entries for Home and About. We will change About to be registered and add two new entries for Dashboard and Logout.
Create Login & Sign-up form
Open up the Home.vue file in the views folder. Delete all the HTML code in the template section. Replace it with this code that provides a very basic login form. Here is the code:
Update the Routes
Currently, the URL to display our Register page is /about. Let’s change that to be /register. Open up the index.js file in the router folder. Change the second route for /about to be /register. Your routes array should look like this:
While we are in this file let’s go ahead and add an entry to display our dashboard component. Add a 3rd route to the display /dashboard. Your routes array should now look like this:
Register New User
Earlier when we updated the About.vue file to register users we had a call to a method called Register. We need to add the functionality to register new users.
First, let’s check out the Firebase documentation on how to create a password-based account. Firebase Auth has a method called createuserWithEmailAndPassword. You need to pass in the user’s email and password. This method will either register the user and return a user object or it will return an error message. Let’s implement this method now.
Open up the About.vue file. We need to add an email and password to our data object. In your script section add the following data object:
Next, add a methods object with one method called to register. We can copy the example from the Firebase documentation for this method. We will need to make the following changes to the code from the documentation:
- We will not use the user object
- Display an alert if login fails
- If the user is registered redirect them to the login page
Here is the code for the register method:
Let’s test registering our first user for our application. Click on Register in the navigation. Enter an email address and password and click the Register button.
Now that we have successfully implemented registering new users for our application, we need to implement the ability for the users to login.
Login Users
We used the code provided by Firebase to register a new user. The Firebase documentation page provides a sample code to log in as a user with an email address and password. The Firebase auth method we will use is signInWithEmailAndPassword.
Like Register, we will make the same changes to the sample code. We will alert the user if they are logged in successfully and redirect them to the Dashboard page.
If login fails, then we display an alert with an error message.
Here is the login method which you should have in your Home.vue file.
Create a Route Guard
We don’t want users to be able to navigate to /the dashboard unless they have logged in. We can do this by adding a route guard for /the dashboard.
Open up the index.js file in the router folder. We will add a meta key to the /register route that will say that authentication is required. Here is the updated route:
Before Vue Router processes a route it has a method called beforeEach. We can check to see if the route requires authentication by checking the meta value.
If authentication is required, we need to be able to check if the user is logged in or not. Luckily there is a currentUser object in Firebase Auth. We will use that to check if the user is logged in or not.
If they are currently logged in then we will display the Dashboard page.
If not we will display an alert telling the user they must be logged in and redirect them to the Home page for them to log in.
Here is the code:
Logout Users
The last thing we need to add to our application is the logout method. Firebase Auth provides a signOut method that we will use.
Open up the App.vue file. We will log out the user. If successful, they will receive an alert and be redirected to the Home page.
If the logout fails we display an alert with the error message and redirect them to the Home page.
Add this code for the logout method:
In the code above, we are using Firebase but we do not have any reference to it in our index.js file. We need to add that. Scroll up to the top of the file where the existing import lines are. Add this line:
Now with that added, you can practice registering a new user. Then log in with that user and verify that you are redirected to the Dashboard page. Then log out and verify you are redirected to the Home page.
Congratulations – you have successfully added Firebase Authentication to your Vue application!
Conclusion
Firebase is a very efficient method of adding authentication to your Vue applications. It allows you to add Authentication without having to write your Backend service and implement Authentication yourself.
You should now have the basic tools you need to build a simple application using Vue.js for the client side and Firebase for data storage and hosting.